Data structures are the building blocks of any program. learn them like your career depends on it.
Arrays, graphs, trees, stacks, queues, linked lists, hash tables, and more.
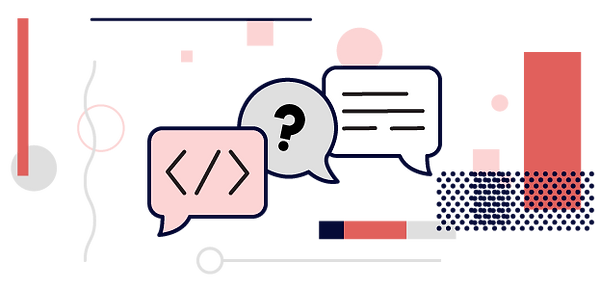
So, you have an interview coming up. You decide it’s a good idea to refresh yourself on data structures. Sure, there are a lot of resources like blogs, videos, short tutorials, even practice questions on sites like Leetcode, but before you know it, you realize there’s way more that you need to cover than you initially thought and you spend more time searching for it than actually studying.
Just as an example, if you had an interview with Google coming up, they put right on their career page that interviewers should, “study up on as many data structures as possible. Data structures most frequently used are arrays, linked lists, stacks, queues, hash-sets, hash-maps, hash-tables, dictionary, trees and binary trees, heaps and graphs. You should know the data structure inside out, and what algorithms tend to go along with each data structure.”
ace your next interview by doubling down on data structures


The process of cracking the coding interview is anxiety-inducing for many developers. There’s so much material to cover, and we’re seeing developers spend their time combing through hundreds of interview questions and not really solidifying what they just learned.
One of the most common points of anxiety that developers face before an interview is: Have I solved enough practice questions? Could I have done more? For a holistic interview preparation study plan, try practicing questions from our curation of LeetCode problems.
Rather than throwing a bunch of interview questions at you and expecting you to memorize them, these data structure courses focus on the vital concepts you need to know for an interview. Throughout are interactive challenges and quizzes to test your understanding, where you can play with real code right from your browser.
In the end, these courses will not only help you nail that interview, but they’ll provide valuable material that’ll be useful at any stage in your career, simply because data structures are essential even if you’re just trying to get better at your current job.
Top 10 Data Structures Interview Questions and Answers
If you have an amazing job opportunity waiting for you, but the grueling interview preparation is shaking your confidence, this article can be very useful. In order to succeed during your interview, you need a quick recap of all the key programming concepts, and one of the most important concepts is data structures. Data structures are the elementary units of a computer program because they are used to organize and manipulate data.
Let's explore the most common data structures interview questions so that you can land your dream job!
Top 10 data structures interview questions
1. What is a data structure?
Data structures are the logical mechanism used to do the following:
-
Organize data
-
Define the relationship between different datasets within a program.
The chosen data structure determines how data can be retrieved for different functions.
2. What are the two major categories of data structures?
There are two main types of data structures:
-
Linear data structures: In a linear data structure, all the data elements are arranged in a sequence. Each element is related to the one before and after — except the first and last elements. Some examples of linear data structures are as follows:
-
Arrays
-
Stacks
-
Linked lists
-
Queues
2. Non-linear data structures: Data elements in a non-linear data structure are not arranged in a particular order. You cannot pass through all the elements in one go. Instead, they are arranged on many levels in any of the following forms:
-
Trees
-
Graphs
-
Tables
-
Sets
3. Why are data structures important?
Data structures are essential for effective software design because they help with the following:
-
Data storage: They define how records are stored in a database management system for effective persistence.
-
Managing resources and services: Core operating systems resources and functions are managed using data structures for memory allocation, file directory management, process scheduling queues, etc.
-
Data exchange: Data structures store information shared between applications like TCP/IP packets.
-
Ordering and sorting: Using different data structures, data can be ordered according to a given priority.
-
Indexing: Complex data structures like B-trees can accelerate data retrieval from database files.
-
Searching: Data structures speed up the search process when finding a target data element from a dataset arranged in a particular structure.
-
Scalability: Big data applications with distributed storage sites require data structures to optimize scalability by replicating the fundamental structure of their data.
These functions make data structures useful for real-time applications like genetics, image processing, blockchain, database management, statistical analysis, AI, simulation, compiler design, and operating systems.
4. Define the heap data structure
A heap is a non-linear data structure in which the tree is completely binary — all its levels are filled, and the last one is as left as possible. There are two types of heap data structures:
-
Max heap: The root node denotes the highest value data element in the binary tree and all its sub-trees recursively.
-
Min heap: The root node contains the minimum data element, which is recursively true for all the sub-trees.
5. What type of data structure is a linked list, and when is it used?
A linked list is a dynamic data structure in which each node contains two fields — a data field and a link field. The randomly stored nodes are connected to their adjacent nodes with a pointer to form a chain-like structure. Depending on the application, it is considered either a linear or non-linear data structure. Linked lists can be useful for the following applications:
-
The implementation of stack, queue, binary trees, graphs, and dynamic memory allocation
-
Real-time computing, like round-robin scheduling, for operating systems tasks
-
Forward and backward operations in a browser
6. What is the difference between a file structure and a storage structure?
The primary difference between a file structure and a storage structure lies in the type of memory area they access.
File structure: File structure data is stored on secondary or auxiliary storage, e.g. a hard disk. This data remains there until manually deleted.
Storage structure: Storage structure data is stored in the RAM and is deleted once its related function is executed.
7. What is a stack data structure and how is it different from a queue?
Stack is a linear data structure that allows data insertion (push operation) and deletion (pop operation) from only the top point. This approach is called either a LIFO (Last-In-First-Out) list or a FILO (First-In-Last-Out) list. A stack data structure can be illustrated using the real-life example of a large pile of laundry where you can only access the clothes at the top of the stack. Stack data structures are useful for solving recursion problems.
A queue data structure is also a linear data structure, but it is based on the FIFO (First In First Out) principle. The REAR end is used for insertion (enqueue), and the FRONT end for deletion (dequeue). Queue data structures can be used, for example, to generate waiting lists for a single shared resource like a printer.
8. What is an array?
An array data structure is an efficient way of storing data because it eases data access. Data is retrieved using an index position as each element in an array is numbered. This means that data can be accessed in any order, though it's entered in order of its creation. Arrays are widely used in databases and other computing systems where large amounts of data need to be frequently accessed.
9. What are multidimensional arrays?
A multidimensional array is made up of more than one array. It is useful for storing data with multiple indexing — such as data elements in tables and image processing.
10. Discuss binary search and its applications
Binary search is an algorithm that efficiently searches for a specific value in a sorted dataset. The data is sorted in either ascending or descending order, and the middle element of this dataset is identified. The target value is then searched in two parts, depending on whether the middle value is higher or lower than the target value. The split and search process continues until the target value is found. This is a much more efficient search algorithm compared to linear search.
Some of its common applications include semiconductor test programs, numerical solutions to equations, and searches for words in dictionaries.
Learn more to ace your interview!
The entire purpose of this list is to give you a compact overview of interview questions for data structures so that you can roughly self-evaluate your skills. You can break down your study plan according to these topics. Still, there is no need to stress-read your older notes and thick textbooks — our courses can refresh your understanding of data structures in no time:
Whether it's C++ data structures or Java data structures interview questions, test yourself with the quizzes and improve your code by learning the implementation of data structures in Playgrounds. And because data structures and algorithm interview questions are closely related, check out the Algorithms for Coding Interviews in Java course to perfect your coding skills.
Find the course that's right for you, start prepping for your interview, and land your dream job.
Start your coding interview prep today.