Top 20 Python Interview Questions (2023)
The rise of Python in the tech industry is hard to ignore. It is simple in nature and can achieve a lot with little code. These qualities make it a top pick to learn machine learning, web development, and more. Big tech companies like Instagram, Spotify, and Netflix all rely on Python. This means that Python developers are in high demand worldwide, with top companies offering great benefits.
As such, Python interviews have become tougher. So, this blog aims to give you a list of commonly asked questions to help you not just get through, but shine in interviews. If you’re aiming for a job at a big tech company or just looking to improve your Python skills, this article is for you.
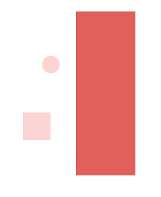
Basic Python Interview Questions
1. What are the key features of Python?
Python boasts the following features:
-
Python is free and open-source: Python is available for free on its official website. Being open-source, its source code is accessible to everyone, allowing you to freely download, use, and share it.
-
Python supports GUI programming: You can create graphical user interfaces using modules like PyQt5, PyQt4, wxPython, or Tk.
-
It supports object-oriented programming: Python is an object-oriented programming language in which objects are associated with both properties and methods.
-
It’s simple to learn to write code in Python: Because Python is a high-level programming language that is simpler to learn, coding in Python is straightforward. Even beginners can grasp its basics quickly. This makes Python developer-friendly.
-
It is a portable language: You can run Python code on any platform, so if something is written on Windows, it can run on other operating systems as well.
-
Python is an interpreted language: This means that the Python program's source code is converted into bytecode, which is subsequently executed by the Python virtual machine. This distinguishes Python from other compiled languages like C and C++, where the code undergoes a build and linking process.
2. Explain the difference between lists and tuples in Python.
The main difference between lists and tuples is that tuples are immutable — they can’t be changed. On the other hand, lists are mutable and can be modified. However, lists are less memory-efficient than tuples.
3. What does PEP 8 mean?
PEP 8 stands for Python Enhancement Proposal 8. It presents coding standards for Python programming. These guidelines help in writing clear, skillfully indented code.
4. What is type conversion in Python?
When you change the value of one data type (like integer, string, float) to another in Python, it’s called type conversion. There are two main type conversion methods: Implicit Type Conversion and Explicit Type Conversion.
5. What is Python Path?
Pythonpath is an environment variable that directs the Python interpreter to locate libraries and applications. It functions the same way as the PATH environment variable in languages like C but includes extra directories for Python modules.
6. What are namespaces in Python?
A namespace is like a dictionary of established symbols paired with details about the objects they point to. The object names serve as keys, and the associated objects represent the values.
Advanced Python Interview Questions
7. When do you use *args and **kwargs in Python?


When you are unsure about the number of keyword arguments to pass to a function, you can use *args and **kwargs as arguments of a function. It should be noted that *args are always used before **kwargs.
8. What is SVM in Python?
Support Vector Machines (SVMs) are supervised learning algorithms used for classification, regression, and detecting outliers. One of their main benefits is their efficiency in handling high-dimensional spaces.
9. Why do you use self in Python?
‘Self’ refers to the instance of the class in Python. Through ‘self,’ we can access the class’s attributes and methods. It associates the attributes with the provided arguments. The need for using ‘self’ arises because Python doesn’t employ the ‘@’ notation for referencing instance attributes.
10. What are Python docstrings?
A docstring is a text description embedded within a Python module, class, function, or method. This way, programmers can understand what the module does without seeing the details of the code itself. Often, these docstrings are used to automatically create online documentation.
11. Why does Python use __ init __?
In Python, the __init__ method is part of a class. Its purpose is to initialize the object’s attributes when you create it. When defining the __init__(self) method, ‘self’ is included as a default parameter in the argument list. This ‘self’ parameter refers to the instance of the class itself. It’s not important to always include an __init__ function, but if you don’t, Python will provide a default constructor for the class.
Python Scripting Interview Questions
12. Define pickling and unpickling.
‘Pickling’ means the conversion of an object hierarchy into a byte stream. Conversely, ‘unpickling’ is the process of transforming a byte stream — either from a binary file or a bytes-like object — back into its original object hierarchy.
13. How is memory managed in Python?
Memory management in Python is handled through a private heap that stores all Python objects and data structures. The memory manager internally oversees the allocation and deallocation within this private heap. Reference counting tracks how often an object is referenced within a program. When this reference count drops to zero, it indicates that the object is no longer in use and is eligible for garbage collection.
14. What is Flask in Python used for?
Flask is a Python web framework that offers a range of handy tools and functionalities for crafting web apps and APIs in Python. It’s ideal for beginners as it allows for rapid development with just a single Python file.
Python OOPS Interview Questions
15. Describe how you can create an empty class in Python.
You can create an empty class using the ‘pass’ statement. The ‘pass’ statement is a placeholder that does not act; it’s essentially a null operation. Despite being empty, you can still create objects from such a class. Take a look at the sample code for it below:
class EmptyClassExample:
pass
object=EmptyClassExample()
object.name="Educative"
print("Name assigned= ", obj.name)
Output: Name= Educative

16. Explain, with an example, how inheritance works in Python.
The concept of inheritance creates a relationship where certain classes inherit attributes and behaviors from a parent class. For instance, consider a parent class named ‘Bird’ and a child class called ‘Parrot.’ In this context, ‘Parrot’ would inherit characteristics from the ‘Bird’ class, making a Parrot a type of Bird. This ability makes it easier to develop and maintain applications.
17. What is polymorphism in Python?
Polymorphism allows us to use the same method in both the parent and the child classes. So, when you have a child class inheriting from a parent class, you can actually tweak the methods you’ve inherited without any fuss.
Python Interview Questions for Data Science
18. What are the commonly used Python libraries for data analysis?
Here are some Python libraries used for data analysis:
-
Numpy and Scipy for fundamental scientific computing
-
Pandas for data manipulation and analysis
-
Matplotlib for plotting and data visualization
-
Scikit-learn for machine learning and data mining
-
Keras for making deep learning models
-
TensorFlow for building AI models
Numpy Interview Questions

19. What is NumPy?
NumPy specializes in array handling. It offers tools for linear algebra, Fourier transforms, matrix operations, standard trigonometric functions, arithmetic operations functions, handling complex numbers, etc.
20. List some uses of NumPy.
Here are some NumPy operations that can help with scientific computing:
-
Vector multiplication
-
Matrix-to-matrix and matrix-to-vector multiplication
-
Performing element-wise calculations on vectors and matrices (like addition, subtraction, multiplication, and division).
Learn data science with Python to enhance your understanding of questions like the ones above.
Start Studying for Your Python Interview Today
You cannot ace the Python interview with just theoretical knowledge — you need to put that knowledge into practice. Whether it’s freelancing or working for big tech companies, these hands-on experiences provide invaluable insights into your weaknesses while honing your skills.
Prepare for tough interview questions with our course “Grokking Coding Interview Patterns in Python.” This intermediate-level course is designed to help you do the following:
-
Understand the patterns behind common Python coding interview questions
-
Evaluate trade-offs in time and space complexity
-
Practice in an in-browser coding environment