Top 10 JavaScript Interview Questions and Answers
JavaScript is one of the most widely used programming languages in the world. According to Wikipedia, 97% of websites use JavaScript for server-side programming. So if you’re a developer or related to the tech industry in any way, you must have used or at least heard of JavaScript.
That being said, there are a lot of JavaScript-related questions that you will likely face during different coding interviews. In particular, if you’re applying for a software developer/engineer position, you’ll see numerous JavaScript-related questions popping up in your interviews.
Whether you’re preparing for an upcoming interview or looking to brush up on your JavaScript expertise, our curated list of the top JavaScript interview questions (and their answers) will help you gain an edge in the competitive job market. Dive in and equip yourself with the knowledge to tackle any JavaScript-centric conversation confidently.
JavaScript Coding Interview Questions for Beginners
If you’re a beginner just stepping into the tech world, having a strong grasp of JavaScript is extremely important. You must practice your JavaScript coding skills to prepare for interviews.
We’ve compiled a list of JavaScript coding interview questions that are usually asked in beginner-level interviews. Don’t worry — we’ve answered them all for you as well. Let’s take a look.
1. What is the difference between Java and JavaScript?
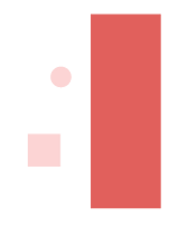
Despite sharing a similar name, Java and JavaScript fundamentally differ in their design, purpose, and application. Java is an object-oriented programming language primarily used for building standalone applications, mobile apps, and large-scale backend systems. Java applications are typically compiled into bytecode, which the JVM executes on the target machine.
In contrast, JavaScript is a lightweight scripting language that helps add interactivity to web pages. Over the years, its role has significantly expanded, and now it powers both client-side and server-side applications, thanks to environments like Node.js. JavaScript is an interpreted language, which means it’s executed line-by-line in real time, unlike Java’s compiled approach.
2. What are callbacks?
A callback is a function that is passed as an argument to another function and is executed after the completion of that function. Callbacks are foundational in JavaScript, especially for asynchronous operations like reading files or making API calls, because they allow functions to run in the background and call the callback once they’re done. This mechanism ensures that certain operations are completed before proceeding, thereby managing the flow of asynchronous tasks within the language.
3. Why is JavaScript single-threaded?
JavaScript is a single-threaded language, which means only one line of code can be executed at one time. This is primarily because of JavaScript’s origins and the nature of its main application: web browsers. When Netscape first created JavaScript in the 1990s, its main purpose was to add interactivity to web pages.
4. What is the use of void (0)?
In JavaScript, void(0) is an expression that returns the undefined value. Historically, it was commonly used in hyperlink href attributes to prevent the browser from navigating to a new page when a user clicked the link. By setting href="javascript:void(0);", developers could ensure that the hyperlink, when activated, would execute JavaScript without triggering a page refresh or navigation.

5. What is the difference between == and === in JavaScript?
-
Double Equals (==): This is the loose equality operator. It compares two values for equality after performing any necessary type of coercion. This means that if the compared values are of different types, JavaScript will try to convert one or both values to a common type before comparing them.
Example: 1 == "1" returns true because the string "1" is implicitly coerced to the number 1 before the comparison.
-
Triple Equals (===): This is the strict equality operator. It compares two values for equality without performing type coercion. If the values are of different types, the comparison will immediately return false. It’s generally recommended to use === over == in most situations in order to avoid unexpected results due to type coercion.
Example: 1 === "1" returns false because no type of coercion is performed, and a number is not strictly equal to a string.
6. What are truthy and falsy values in JavaScript?
A value is considered “truthy” if it evaluates to true in a Boolean context; a value is “falsy” if it evaluates to false. The following are the primary falsy values in JavaScript:
-
false
-
0 and -0
-
"" (empty string)
-
null
-
undefined
-
NaN
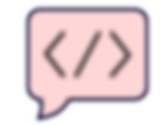
7. Explain the concept of hoisting in JavaScript.
Hoisting in JavaScript is a behavior in which variable and function declarations move to the top of their containing scope during the compilation phase before the code is executed. This means that variables and functions can be used before they are declared in the code.
Advanced JavaScript Interview Questions
8. Explain the concept of closures in JavaScript.
Closures in JavaScript refer to the ability of a function to remember and access variables from an outer (enclosing) scope, even after the outer function has finished executing. Closures are created whenever a function is defined inside another function, with the inner function referencing variables from the outer function. This behavior allows for data encapsulation and private data patterns in JavaScript.
9. What is the prototype chain, and how does prototypal inheritance work in JavaScript?
The prototype chain in JavaScript is a mechanism that allows objects to inherit properties and methods from other objects. Every object in JavaScript has a hidden property called [[Prototype]] — often accessible via the __proto__ property or Object.getPrototypeOf() — which points to another object, the “prototype.”
Prototypal Inheritance:
In JavaScript, inheritance is based on prototypes. When one object inherits from another, it essentially sets its prototype to point to the parent object. When a property is not found on the child object, the prototype chain can be traversed to find the property on the parent object (or further up if necessary).
10. Explain the differences between Promises and async/await.
Promises: Introduced in ES6, a Promise represents a future value — either a resolved value or a reason for rejection. Promises have three states: pending, resolved (fulfilled), or rejected. Promises are usually used with .then() for resolved values and .catch() for errors.
async/await: Introduced in ES8 (or ES2017), async/await is syntactic sugar on top of Promises, designed to make asynchronous code look and behave more like synchronous code. An async function always returns a promise, and inside an async function, you can use the await keyword to pause the function’s execution until the promise is resolved, making it easier to read and write asynchronous code.
Ace your JavaScript Coding Interview!
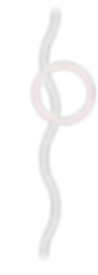
Navigating the maze of a JavaScript coding interview can be intimidating. But with the right preparation, conceptual clarity, and practice, you can stand out from the crowd. These 10 JavaScript interview questions and answers can help you practice, but they are not enough to ace your coding interview.
If you want to practice thoroughly and get the job you want, then we suggest “Master the JavaScript Interview” by Educative. The course includes the following:
-
Twenty curated interview questions from experts
-
Multiple solutions for each question
-
Advanced ways of manipulating data, which is something that interviewers look for.