Have you ever found yourself rushing to learn a new programming language just weeks before a coding interview? It can be a stressful experience, but it’s a challenge that many developers face. Whether you’re switching from Python to Java or transitioning from JavaScript to Go, with the right approach, mastering a new language in time for a technical interview is entirely within reach.
In this blog, we’ll guide you on how to learn and adapt to a new programming language from a technical perspective. We’ll cover key concepts such as understanding language syntax, exploring standard libraries, and implementing data structures and algorithms. By focusing on these technical aspects and utilizing available resources, you can build your skills and gain the confidence needed to excel in coding interviews.
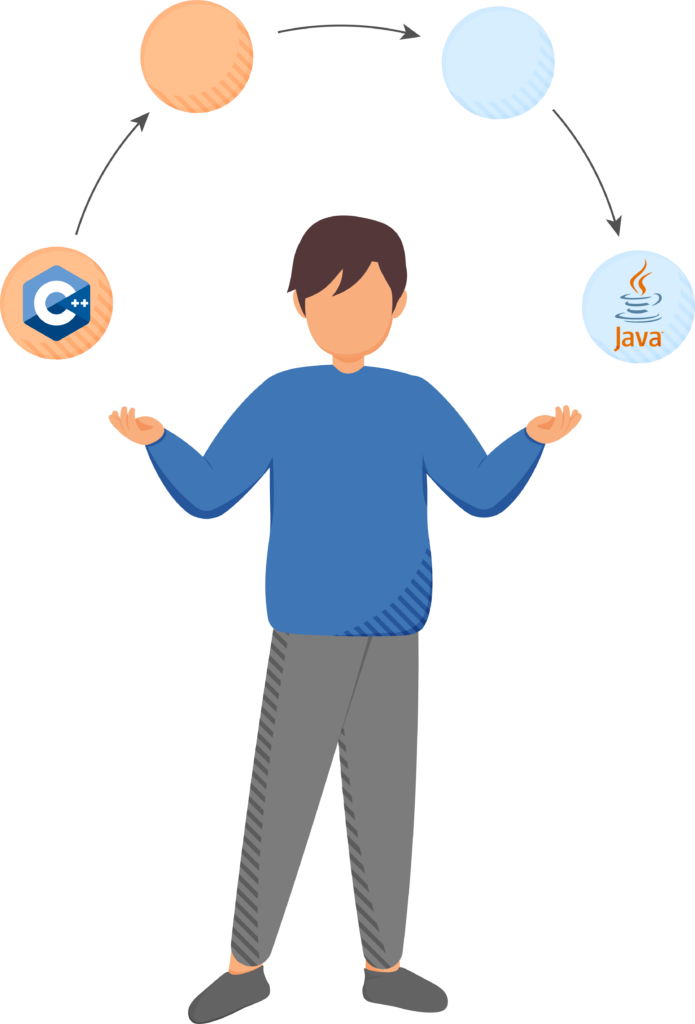
Choose the right programming language
Before diving into preparation, ensure that the new programming language you’re learning aligns with the types of companies and roles you’re targeting. For example, Python, Java, and C++ are often preferred for algorithmic interviews, while languages like JavaScript might be more common for front-end roles.
Below are some suggestions to help you choose the right language:
- Research the language preferences of the companies you’re applying to.
- Consider the industry standards and what language best suits the job requirements.
- Choose a language that has strong community support and extensive learning resources.
Once you’ve chosen the right language, it’s time to dive into the core components that will prepare you for interviews.
Master the fundamentals of your new language
Understanding the core aspects of the new language is important. This includes:
Syntax and semantics: Begin by learning the basic syntax of the new language. This involves understanding how to declare variables, write loops, define functions, and handle exceptions. Each language has its unique way of handling these basics; mastering them is the first step toward becoming proficient.
Mastering the syntax of a new programming language can be challenging, but understanding the similarities and differences between the new language and the ones you’re already familiar with can simplify the process. Below is a comparison of key programming constructs—like variable declarations, loops, functions, and exception handling—across popular languages:
Language | Variable | Loop Syntax Example | Function Definition | Exception Handling |
---|---|---|---|---|
Python | x = 10 | for i in range(5): | def func(): | try: … except: |
Java | int x = 10; | for (int i=0; i<5; i++) | void func() {} | try { … } catch (Exception e) {} |
C++ | int x = 10; | for (int i = 0; i < 5; i++) | void func() {} | try { … } catch (exception& e) {} |
JavaScript | let x = 10; | for (let i = 0; i < 5; i++) | function func() {} | try { … } catch (e) {} |
Go | x := 10 | for i := 0; i < 5; i++ | func func() {} | try { … } catch (e) {} |
C# | int x = 10; | for (int i=0; i<5; i++) | void Func() {} | try { … } catch (Exception e) {} |
Standard library: Familiarize yourself with the standard library of the new language. The standard library provides a wealth of pre-written code that can save you time and effort. Knowing which functions and classes are available can greatly enhance your productivity.
Below is a comparison of commonly used library functions across popular languages:
Language | Common Library Functions |
---|---|
Python | math , collections , itertools |
Java | java.util , java.io , java.lang |
C++ | vector , algorithm , map |
JavaScript | Array , Math , Date |
Go | fmt , math , sort |
C# | System , System.Collections.Generic |
Below are some code examples of using these libraries in different languages:
Python
In the following code, the math
module is used to perform mathematical operations, such as calculating the square root of 16, which gives 4.0
. The collections
module’s Counter
class counts the occurrences of each character in the string 'aabbcc'
, resulting in a dictionary-like output showing the frequency of each character.
Use of library in Python
Java
The following code imports the ArrayList
class from Java’s java.util
package and creates an ArrayList
of integers. It adds the numbers 1
and 2
to the list and then prints the list, resulting in the output [1, 2]
.
Use of library in Java
C++
The following code initializes a vector
with the values {1, 3, 2}
, sorts the vector in ascending order using std::sort
, and then prints the sorted values 1 2 3
to the console.
Use of library in C++
JavaScript
The following code creates an array arr
, sorts it, and prints the sorted array [1, 2, 3]
. It also creates a Date
object representing the current date and prints it in a human-readable format, like “Tue Aug 14 2024”.
Use of library in JavaScript
Go
The following code uses Go’s math
package to calculate 2
raised to the power of 3
and prints the result, which is 8
. The fmt.Println
function is used to display the output to the console.
Use of library in Go
C#
The following code creates a list of integers in C#, sorts the list in ascending order, and then prints the sorted list as a comma-separated string using Console.WriteLine
. The output is 1, 2, 3
.
Use of library in C#
Data types and structures: Understand the basic data types and structures used in the language. This includes common structures such as arrays, lists, sets, and maps. Each language has its way of implementing these structures, and understanding them is key to writing efficient code.
Below is a comparison of these common data structures across popular languages:
Language | Array | List/Vector | Set |
---|---|---|---|
Python | list | list | set |
Java | int[] | ArrayList | HashSet |
C++ | int arr[] | vector<int> | set<int> |
JavaScript | Array | Array | Set |
Go | [...]int | []int (slice) | map[int]struct{} |
C# | int[] | List<int> | HashSet<int> |
Translate data structures and algorithms
One effective way to learn a new language is to implement familiar data structures and algorithms in the new language:
- Data structures: Implement basic data structures such as linked lists, stacks, and queues. This will deepen your understanding of the language’s syntax and its approach to memory management and data manipulation.
- Sorting algorithms: Start by writing implementations of common sorting algorithms. This will help you understand the language’s syntax and how it handles loops and conditionals.
If you’re looking for a structured approach to mastering data structures for coding interviews in a new language, Educative offers courses like “Data Structures for Coding Interviews,” available in Python, Java, and more.
Practice coding problems regularly
Practice problems in the new language and learn from your mistakes to improve your understanding. Below are some tips to help you start practicing problems in the new language:
- Start with familiar concepts: Begin by solving problems you already know in your previous language. This helps you focus on learning the new language’s syntax and features without getting overwhelmed.
- Increase difficulty gradually: Start with easy problems to grasp the basics, then move to more complex challenges. This progression builds confidence while deepening your understanding of the new language.
- Use previous knowledge: Draw parallels between the new language and your proficiency. For example, if you know Python’s list comprehensions, explore similar features in the new language to accelerate learning.
Write idiomatic and clean code
Understanding and following language-specific idioms and best practices will help you write effective and readable code:
- Learn language-specific idioms: Each programming language has its idiomatic way of solving problems. Understanding these idioms can make your code more efficient and readable.
- Python: It emphasizes simplicity and readability, often using list comprehensions and generator expressions.
- Java: It follows object-oriented principles, with a focus on using classes and objects.
- JavaScript: It utilizes functional programming aspects and handles asynchronous programming with promises and async/await.
- C++: It emphasizes RAII (Resource Acquisition Is Initialization) for resource management and extensively uses the Standard Template Library (STL) for efficient data manipulation.
- Go: It prioritizes simplicity and concurrency, using goroutines for concurrent programming and
defer
for clean resource management. - C#: It uses LINQ for querying collections in a declarative style and utilizes async/await for asynchronous programming, following object-oriented and component-based principles.
Each programming language has its own design focus and idiomatic features that reflect its strengths. Understanding these core principles can help you write more idiomatic and efficient code as you transition to a new language. Below is a comparison of the primary focus and key idiomatic features of several popular languages:
Language | Focus | Key Idiomatic Features |
---|---|---|
Python | Simplicity and readability | List comprehensions, generator expressions |
Java | Object-oriented principles | Classes and objects, Stream API |
JavaScript | Functional programming and asynchronous handling | Arrow functions, promises, async/await |
C++ | Resource management and efficiency | RAII, Standard Template Library (STL) |
Go | Simplicity and concurrency | Goroutines, defer for resource management |
C# | Object-oriented and component-based principles | LINQ, async/await, auto-implemented properties |
- Adhere to style guides: Follow the language’s style guide, such as PEP 8 for Python or the Google Java Style Guide for Java. These guides provide conventions for writing clean and readable code.
- Ensure modularity: Write modular code with well-defined functions and classes. This practice makes your code easier to understand, test, and maintain.
- Use comments and documentation: Use comments and documentation to explain complex logic. This will help others understand your code and make it easier for you to revisit and modify your code in the future.
Review and refactor your code
Regular code review and refactoring are important for maintaining and enhancing the quality of your code:
- Peer reviews: Participate in code reviews to get feedback from others. Peer reviews can provide new perspectives and highlight areas for improvement that you might have missed.
- Self-review: Periodically revisit your solutions to improve and optimize them. Look for more efficient algorithms, better use of language features, or clearer code structure.
Practice mock interviews in the new language
Simulate real interview conditions to build your confidence:
- Platforms: Use mock interview platforms like Educative to practice with real interviewers. Go ahead and check out our mock interviews . It will take you to the mock interviews page, where you’ll find a bundle of interviews in different languages.

- Peer practice: Conduct mock interviews with friends or colleagues. This practice can help you become more comfortable expressing your thought process and explaining your solutions.
- Iterative improvement: Take feedback and refine your approach based on the feedback you receive.
Utilize online resources
There are multiple online resources available to help you learn a new language. Below are some resources that can assist you in preparing for coding interviews in a new language:
- Online courses and tutorials: Platforms like Educative offer structured courses tailored to various programming languages. These courses can provide a guided learning path, helping you cover all important topics structurally.
- Coding platforms: Websites like Educative allow you to practice coding problems in different languages. These platforms provide immediate feedback and often include discussions on various solutions, which can be highly educational.
- Official documentation: Go through the official documentation of the language. It is the most authoritative source of information and often contains tutorials and examples. For Python, check the Python Docs ; for Java, refer to the Java Docs; for C++, consult the C++ Reference. JavaScript is well-covered by the MDN Web Docs, Go has its Go Docs, and C# documentation can be found in the C# Docs.
Stay updated
Programming languages and technologies evolve rapidly, making it important to stay informed about the latest developments and advancements:
- Community engagement: Join forums, follow influential developers, and participate in language-specific communities. Engaging with the community can provide valuable insights and keep you informed about the latest trends and best practices.
- Continuous learning: Regularly read blogs, watch tutorials, and take courses to stay updated with the latest features, best practices, and industry trends. Continuous learning ensures that you remain proficient and competitive in your field.
Conclusion
Transitioning to a new programming language for coding interviews doesn’t have to be overwhelming. By mastering the fundamentals, practicing with real problems, and using the right resources, you can gain confidence and proficiency in record time. Remember, consistent practice and persistence are key to mastering any language—so dive in, and get coding!
If you’re looking for good resources to help you practice real coding interview problems in the programming language of your choice, take a look at these: