If you’re preparing for an interview at Lyft, you’re likely experiencing a mix of excitement and nervousness. Landing a job at a well-known company like Lyft is a significant opportunity, whether you’re applying for a role in software engineering, product management, data science, or another department. Success lies in thorough preparation, strategic practice, and a deep understanding of Lyft’s interview structure.
Lyft’s interview process is designed to assess your technical expertise, problem-solving abilities, system design knowledge, and cultural fit. Candidates must be well-prepared for coding challenges, system design discussions, behavioral questions, and real-world problem-solving scenarios.
This guide will walk you through different interview categories, share sample questions, and suggest resources to help you ace your Lyft interview. Additionally, we’ll recommend an AI-powered mock interview platform to enhance your preparation.
Technical interview questions
Technical interviews at Lyft test your coding skills, problem-solving ability, and algorithmic thinking. Expect questions on data structures, algorithms, and system efficiency.
Some recently asked coding questions at Lyft are listed below:
1. Rotting oranges
Problem: Given an \( m × n \) grid where each cell can have one of three values:
- 0: An empty cell
- 1: A cell containing a fresh orange
- 2: A cell containing a rotten orange
Any fresh orange 4-directionally adjacent to a rotten orange will become rotten at each minute.
Determine the minimum time required for all fresh oranges to rot. If all oranges can’t rot, return -1.
Why, Lyft might ask: This problem tests your ability to simulate real-time events, like dispatching drivers and tracking status updates in a grid-based service area.
Solution: We use breadth-first search (BFS) to simulate the rotting process, starting from all initially rotten oranges. Each level of BFS represents a minute, where adjacent fresh oranges rot. We continue this process until no fresh oranges remain or no further spread is possible. If all fresh oranges are rotted, we return the total time taken; otherwise, we return -1
if some remain unreachable.
Rotting oranges
Time complexity: \( O(n \times m) \)
Space complexity: \( O(n \times m) \)
2. Max Stack
Problem: Design a max stack data structure that functions like a regular stack but also efficiently supports retrieving and removing the maximum element in the stack.
Class implementation:
- MaxStack(): Initializes an empty stack.
- push(x: int) -> None: Pushes the element
x
onto the stack. - pop() -> int: Removes and returns the top element of the stack.
- top() -> int: Retrieves the top element without removing it \( O(1) \) time complexity).
- peekMax() -> int: Retrieves the maximum element in the stack without removing it.
- popMax() -> int: Retrieves and removes the maximum element from the stack. If multiple occurrences exist, only the topmost one is removed.
The solution should ensure:
- \( O(1) \) time complexity for top().
- \( O(log n) \) time complexity for push(), pop(), peekMax(), and popMax().
Why, Lyft might ask: It’s perfect for testing your understanding of stack operations with extra constraints. This is applicable to ride history or fare tracking, where you might need “max” values on the fly.
Solution: We use two stacks: one to store all elements and another to keep track of the maximum values. When an element is pushed, it is added to both stacks, ensuring the max stack always has the highest value at each level. When popping, elements are removed from both stacks to maintain consistency. Retrieving the maximum is done in constant time by checking the top of the max stack. To remove the maximum element, elements are temporarily stored until the max is found and removed, then the stack is reconstructed.
Max Stack
Time complexity:
- \( O(1) \) time complexity for top().
- \( O(log n) \) time complexity for push(), pop(), peekMax(), and popMax().
Space complexity: \( O(n) \)
3. Water and jug problem
Problem: You have two jugs with capacities of x
liters and y
liters, along with an unlimited water supply. Your goal is to determine if you can measure exactly target
liters using the following actions:
- Fill either jug to its maximum capacity.
- Empty either jug completely.
- Pour water from one jug into the other until the receiving jug is full or the transferring jug is empty.
Return true if it is possible to obtain the exact target amount of water; otherwise, return false.
Why Lyft might ask: This tests your understanding of number theory, state transitions, and problem-solving under resource constraints—similar to optimizing limited driver availability or resource pooling.
Solution: Use Bézout’s theorem, which says the problem is solvable if the target is a multiple of the GCD of the jug sizes. We simulate all possible pouring actions using BFS or DFS to check if the target can be reached.
Water and Jug Problem
Time complexity: \( O(log (\min(x,y))) \)
Space complexity: \( O(log (\min(x,y))) \)
4. Time-based key-value store
Problem: Design a time-based key-value data structure that allows storing multiple values for the same key at different timestamps and retrieving the most recent value for a given key at or before a specified timestamp.
- TimeMap(): Initializes the data structure.
- set(key: str, value: str, timestamp: int) -> None: Stores the given
key
withvalue
at the specifiedtimestamp
. - get(key: str, timestamp: int) -> str: Retrieves the most recent value associated with
key
at or beforetimestamp
. If no such value exists, returns""
.
Why, Lyft might ask: Tracking ride statuses, driver availability, and surge pricing historically fits this problem‘s structure perfectly.
Solution: Store a list of (timestamp, value) pairs for each key. Use binary search on the timestamp list to efficiently find the latest value before or at the given timestamp in \( O(\log n) \) time.
Time-Based Key-Value Store
Time complexity:
- set(key, value, timestamp) = \( O(1) \)
- get(key, timestamp) = \( O ( \log n ) \), where n is the number of timestamp-value pairs associated with the key.
Space complexity: \( O(n) \), where \( n \) is the total number of (timestamp, value) pairs stored in the data structure.
5. Range sum query 2D - Immutable
Problem: Design a NumMatrix data structure that efficiently handles multiple sum queries on a 2D matrix. Given a matrix, the goal is to compute the sum of elements within a specified rectangular subregion, defined by its top-left corner (row1, col1)
and bottom-right corner (row2, col2)
, in \( O(1) \) time.
Class implementation:
- NumMatrix(matrix: List[List[int]]): Initializes the object with the given
matrix
. - sumRegion(row1: int, col1: int, row2: int, col2: int) -> int
- Returns the sum of elements within the rectangular area bounded by
(row2, col2)
and(row2, col2)
. - The implementation should ensure that sum queries run in \( O(1) \) time complexity.
- Returns the sum of elements within the rectangular area bounded by
Why, Lyft might ask: Efficient region-based querying is vital for analyzing large-scale data like city zones, ride demand heatmaps, and fare calculations.
Solution: Precompute a prefix sum matrix where each cell stores the sum from the origin to that point. This allows us to compute the sum of any submatrix in \( O(1) \) time using inclusion-exclusion.
Range Sum Query 2D - Immutable
Time complexity:
- Constructor: \( O(m \times n) \)
- sumRegion(row1, col1, row2, col2): \( O(1) \)
Space complexity: \( O(m \times n) \)
Additional common problems Lyft asks
- Merge k Sorted Lists: Given
k
sorted linked lists, merge them into one sorted list in an efficient manner. - Detect a Cycle in a Linked List: Implement an algorithm to determine if a linked list has a cycle, and if so, find the starting node of the cycle.
- Find the Longest Consecutive Sequence in an Array: Given an unsorted array, find the length of the longest consecutive sequence in \( O(1) \)time.
- Serialize and Deserialize a Binary Tree: Design an algorithm to convert a binary tree into a string format and efficiently reconstruct the tree from that string.
- Courses like Grokking the Coding Interview and Grokking Data Structures and Algorithms help you master crucial coding patterns and problem-solving techniques.
System Design interview questions
Lyft’s system design interviews assess your ability to create scalable, efficient, and reliable systems that handle real-world, high-volume ride data.
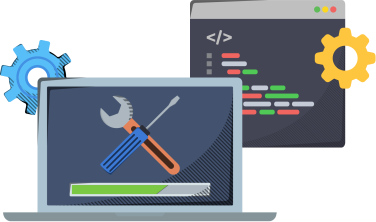
1. Design Lyft’s ride matching system
Key features:
- Match riders with nearby drivers.
- Prioritize driver availability and proximity.
- Handle millions of concurrent requests.
Design highlights:
- Use geohashing to cluster drivers and riders.
- Apply priority queues to optimize matches.
- Store active rides in Redis for real-time lookup.
2. Design a surge pricing system
Key features:
- Adjust ride prices based on demand and supply.
- Handle regional surges dynamically.
Design highlights:
- Use Kafka to stream ride requests.
- Apply sliding window algorithms for real-time demand calculations.
- Update pricing in real-time using distributed caches.
3. Design a driver rating system
Key features:
- Calculate average driver ratings.
- Prevent rating manipulation.
Design highlights:
- Use weighted averages.
- Apply anomaly detection to flag suspicious reviews.
4. Designing a load balancer
Develop a system that efficiently distributes incoming network traffic across multiple servers to optimize performance and reliability.
Key features:
- Dynamically balance traffic based on requests per second (RPS).
- Conduct regular health checks to detect and exclude unresponsive servers.
- Support horizontal scaling to accommodate increasing traffic demands.
Design highlights:
- Implement load balancing algorithms such as Round Robin or Least Connections for effective traffic distribution.
- Leverage tools like NGINX, HAProxy, or cloud-based load balancers for deployment.
- Utilize consistent hashing to ensure a more stable and even traffic distribution.
5. Design a real-time ETA prediction system
Key features:
- Provide accurate estimated time of arrival (ETA) for riders.
- Continuously update ETAs based on traffic, weather, and driver location.
- Scale to handle millions of concurrent users.
Design highlights:
- Use real-time GPS tracking and historical traffic data for predictions.
- Implement machine learning models to improve ETA accuracy over time.
- Store and process location data efficiently using Apache Flink or Google BigQuery.
More questions
- Optimize a dynamic pricing algorithm: Develop a system that adjusts ride prices dynamically based on demand, driver availability, and external conditions like weather or events.
- Develop a real-time location tracking system: Architect a system that continuously updates and displays the live location of drivers and riders during a trip.
- Optimize a route recommendation system: Design a system that suggests the most efficient routes for drivers based on traffic, road conditions, and real-time ride requests.
- Implement a payment processing system for rides: Build a secure and scalable system for handling ride payments, refunds, and fare adjustments with minimal latency.
- Build a safety and trust monitoring system: Design a system that detects and flags unusual ride patterns, driver behavior, or safety concerns to improve rider and driver security.
- System Design interviews at Lyft assess your ability to architect scalable, efficient, and reliable systems. These interviews focus on designing distributed systems, making trade-offs, and solving high-level technical challenges—key concepts covered in "Grokking the Modern System Design Interview."
Behavioral interview questions
Behavioral interviews at Lyft focus on your ability to collaborate, innovate, and solve complex problems in high-stakes environments.
Example questions
- Tell me about a time you solved a difficult problem.
Example answer: During peak hours, ride matching delays increased. I optimized the algorithm to prioritize proximity and demand clusters. This improved match efficiency by 40%, reducing wait times significantly. - Describe a conflict within your team and how you handled it.
Example answer: The frontend and backend teams disagreed on API structure. I facilitated a discussion, ensuring both sides understood the constraints and needs. We implemented a compromise solution, improving collaboration. - Share an experience leading a project under tight deadlines.
Example answer: A third-party provider unexpectedly changed Lyft’s map integration. I led a cross-functional sprint, coordinating teams to redesign and deploy within a week, ensuring a seamless transition for users. - How do you advocate for your ideas?
Example answer: I proposed a batch-processing system to optimize ride completion reports. To gain buy-in, I built a working prototype demonstrating efficiency. Leadership approved, and it reduced processing time by 30%. - Describe how you handle feedback.
Example answer: A senior engineer suggested optimizing API responses for better performance. I reduced payload size, improving app load times. The update enhanced responsiveness and user experience. - How do you ensure inclusivity and diversity in your work?
Example answer: I introduced rotating leadership roles in meetings to amplify all voices. This ensured remote team members and quieter colleagues were heard. The approach strengthened team engagement and collaboration.
More questions
- Describe a situation where you took full responsibility for a complex project and led it to success.
- Tell me about a time you successfully met a critical deadline despite facing significant obstacles.
- Give an example of how you went above and beyond to improve the customer experience.
- Share when you had to quickly adapt to a major shift in your role, team structure, or company strategy.
- For tailored guidance on Lyft Leadership Principles and behavioral questions, explore Grokking the Behavioral Interview, which provides real-world examples to refine your responses effectively.
General tips to ace your Lyft interview
- Know Lyft’s products: Explore Lyft rentals, bikes, scooters, and business offerings.
- Understand Lyft’s business model: Ridesharing, partnerships, advertising, and more.
- Practice behavioral questions: Show empathy, innovation, and impact.
- Sharpen technical skills: Focus on algorithms, system design, and scalability.
- Follow industry trends: Stay updated on ridesharing competitors and market changes.
- Ask thoughtful questions: Dive into Lyft’s roadmap, challenges, and team culture.
Refine your skills with AI-powered mock interviews
To boost your chances of success, practice with AI-driven mock interview platforms. These platforms provide realistic coding, system design, and behavioral interview simulations, offering instant feedback to improve your performance.
Recommended resources
- Grokking the Low-Level Design Interview Using OOD Principles: A battle-tested guide to Object Oriented Design Interviews – developed by FAANG engineers. Master OOD fundamentals & practice real-world interview questions.
- Grokking the Product Architecture Design Interview: The essential guide to API Design & Product Design Interviews – developed by FAANG engineers. Master product design fundamentals & get hands-on with real-world APIs.
Conclusion
Landing a role at Lyft requires a strong foundation in coding, system design, and behavioral skills. To succeed, focus on solving real-world scalability challenges and designing user-centric solutions. For mid-to-senior roles, system design expertise is essential, as you’ll need to demonstrate your ability to architect scalable and efficient systems. In behavioral interviews, Lyft values collaborative leadership and an inclusive mindset, so be ready to showcase how you thrive in a team-driven environment.
To sharpen your skills, leverage platforms like LeetCode for coding practice, Educative for system design courses, and mock interviews to refine your communication and problem-solving strategies. You’ll be well-equipped to ace your Lyft interview and accelerate your career with consistent practice, a strategic mindset, and confidence.
Best of luck on your journey to Lyft!
Frequently Asked Questions
What technical topics should I focus on for the Lyft interview?
Prepare for questions about data structures, algorithms, system design, and scalability. Topics like graphs, dynamic programming, and real-time processing are commonly tested.
How does Lyft assess system design skills?
Lyft evaluates candidates for designing scalable, real-time systems for ride-matching, ETA prediction, and surge pricing. Understanding distributed computing, caching, and database sharding is crucial.
What behavioral questions does Lyft ask?
Lyft focuses on collaboration, innovation, and problem-solving. Expect questions on conflict resolution, leadership, and handling feedback using the STAR method for structured responses.
How can I practice for the Lyft interview?
Use platforms like LeetCode, Educative, and mock interview tools. AI-powered interview simulators can help refine coding and behavioral responses.
What’s the best way to stand out in the Lyft interview?
Show strong problem-solving skills, a deep understanding of Lyft’s business model, and a user-centric mindset. Asking insightful questions about Lyft’s future and team dynamics also leaves a great impression.
Company Interview Questions