If you’re preparing for an interview at X (formerly Twitter), you’re likely feeling excitement and nerves. Solid preparation is the key to success, whether you’re aiming for a role in software engineering, product management, or data science. Twitter’s interview process is well-structured and designed to assess technical expertise, system design knowledge, and cultural fit.
This guide will cover each interview category, provide sample questions, and recommend resources to help you crack your Twitter interview. Also, we’ll suggest an AI-powered mock interview platform to refine your skills.
Technical interview questions
Technical interviews at Twitter test your coding skills, problem-solving ability, and algorithmic thinking. Expect questions on data structures, algorithms, and system efficiency. Below are examples of technical questions.
1. Multiply strings
Problem: Given two non-negative integers, num1
and num2
, represented as strings, return their product as a string.
You cannot use any built-in BigInteger
library. You cannot directly convert the strings to integers (like using int()
in Python).
Solution: We multiply two non-negative integers as strings by simulating manual digit-by-digit multiplication. A result array is initialized to store intermediate values, sized to fit the full product. Digits are multiplied from right to left, with sums added to the correct positions and carry-over handled immediately. Finally, the result array is converted to a string, leading zeros are removed, and the product is returned without using built-in integer conversions.
Multiply strings
Time complexity: \( O (n + m) \), where n
is the length of num1
and m is the length of num2
.
Space complexity: \( m \times n \)
2. Insert delete GetRandom O(1)
Problem: Design a data structure that supports all of the following operations in average \( O(1) \) time:
- insert(
val
): Inserts an itemval
into the set if not present. - remove(
val
): Removes an itemval
from the set if present. getRandom()
: Returns a random element from the current set of elements. Each element must have the same probability of being returned.
Solution: To achieve \( O(1) \) time complexity for insert
, remove
, and getRandom
operations, we can use a combination of a list and a dictionary:
- List: Stores the elements, allowing \( O(1) \) access for the
getRandom
operation. - Dictionary: Maps each element to its index in the list, enabling \( O(1) \) time complexity for
insert
andremove
operations.
Insert delete GetRandom O(1)
Time complexity: \( O(1) \)
Space complexity: \( O(1) \)
3. Tweet counts per frequency
Problem: Implement the TweetCounts
class:
- TweetCounts(): Initializes the object.
- recordTweet(String tweetName, int time): Stores the tweetName at the recorded time (in seconds).
- getTweetCountsPerFrequency(String freq, String tweetName, int startTime, int endTime): Returns an array of the number of tweets for the given tweetName per each time interval. The frequency
freq
can be “minute,” “hour,” or “day,” representing time intervals of 60 seconds, 3600 seconds, and 86400 seconds respectively.
Solution: We use a dictionary (hash map) to store tweet names as keys and a list of timestamps as values, allowing quick lookups and efficient storage. When recording a tweet, we simply append its timestamp to the list, making it an \( O(1) \) operation. For retrieving tweet counts per frequency, we divide the time range into fixed intervals based on the given frequency—minute (60s), hour (3600s), or day (86400s)—and create a list to store counts for each interval. Using binary search, we efficiently locate timestamps within the given range and count occurrences in each interval.
Tweet counts per frequency
Time Complexity:
- recordTweet: \( O(n) \)
- getTweetCountsPerFrequency: \( O(k log n) \)
- recordTweet: \( O(n) \)
- getTweetCountsPerFrequency: \( O(k) \)
4. Design Twitter
Problem: Design a simplified version of Twitter where users can post tweets, follow/unfollow another user, and see the 10 most recent tweets in the user’s news feed. Implement the Twitter
class:
- postTweet(int userId, int tweetId): Compose a new tweet.
- getNewsFeed(int userId): Retrieve the 10 most recent tweet IDs in the user’s news feed. Each item in the news feed must be posted by users who the user followed or by the user themselves. Tweets must be ordered from most recent to least recent.
- follow(int followerId, int followeeId): Follower follows a followee.
- unfollow(int followerId, int followeeId): Follower unfollows a followee.
Solution: We use a dictionary (hashmap) to store the following relationships, mapping each user to a set of users they follow, ensuring efficient lookups for feed generation. We maintain a global timestamp for tweets to track tweet order and store tweets in a dictionary where each user maps to their list of posted tweets. When retrieving the news feed, we gather tweets from the user and their followers, then use a min heap to efficiently retrieve and sort the 10 most recent tweets in descending order.
Design Twitter
5. Best meeting point
Problem: Given a 2D grid of values 0 or 1, where each 1 marks the home of someone in a group, find the minimal total travel distance to a meeting point. The total travel distance is the sum of the distances between the group members’ homes and the meeting point.
Solution: We use the mathematical property that the median minimizes the sum of absolute deviations. First, we collect the row and column indexes of all 1s (homes) in the grid separately. As the optimal meeting point lies near the median, we find the median row and median column from these collected indexes. Finally, we compute the total Manhattan distance, the sum of absolute differences between each home’s and median coordinates.
Best meeting point
Time complexity: \( O(m * n ) \), where m
is the number of rows and n
is the number of columns.
Space complexity: \( O(k) \), where k
is the number of 1
s in the grid.
Additional problems
1. Flatten Nested List Iterator
Problem: You are given a nested list of integers NestedInteger
. Each element is either an integer or a list whose elements may also be integers or other lists. Implement an iterator to flatten it.
NestedIterator
class:
- NestedIterator(List<NestedInteger> nestedList): Initializes the iterator with the nested list.
- next(): Returns the next integer in the nested list.
- hasNext(): Returns true if some integers are still in the nested list and false otherwise.
2. Kth largest element in an array
Problem: Given an unsorted array of integers, find the kth largest element. You may use algorithms like QuickSelect for average-case O(n)
time or a Min Heap of size k
for O(n log k)
time.
3. Cycle detection in a linked list
Problem: Given a linked list, determine if it contains a cycle. Implement the solution using Floyd’s Tortoise and Hare algorithm, which uses two pointers moving at different speeds to detect cycles with O(n)
time and O(1)
space.
4. Merge K sorted linked lists
Problem: Given an array of k
sorted linked lists, merge them into a single sorted linked list. Achieve an efficient solution using a priority queue (min-heap) to always select the smallest current node from the lists, with a time complexity of O(N log k)
, where N
is the total number of nodes.
5. LRU (Least recently used) Cache
Problem: Design and implement an LRU Cache with a fixed capacity that supports get()
and put()
operations in O(1)
time. Use a combination of a HashMap for quick lookups and a Doubly Linked List to keep track of the usage order of elements.
6. Longest substring without repeating characters
Problem: Given a string, find the length of the longest substring that contains no repeating characters. Use the Sliding Window technique with a HashSet or HashMap to achieve an optimal solution with O(n)
time complexity.
7. URL shortener design
Problem: Design a URL shortener system that converts long URLs into shorter ones and allows redirecting from the short URL to the original. Use Base62 encoding (including uppercase and lowercase letters and digits) and a hashing mechanism to generate unique codes while handling collisions and ensuring scalability.
- Courses like Grokking the Coding Interview and Grokking Data Structures and Algorithms help you master crucial coding patterns and problem-solving techniques.
System Design interview questions
Twitter’s system design interviews assess your ability to design scalable, efficient, and distributed systems. Expect questions on architecture, trade-offs, and best practices.
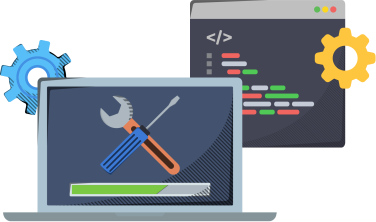
Below are examples of system design questions.
Problem: How would you design a system that efficiently stores and retrieves tweets for millions of users in real time?
Key features:
- Efficient storage of tweets
- Fast retrieval of tweets for a given user
- Handling trending tweets
Design highlights:
- Use NoSQL databases like Cassandra for high-write scalability.
- Implement sharding and replication to handle large data loads.
- Use Redis or Memcached for fast retrieval of recent tweets.
Problem: How would you design a rate limiting system to prevent excessive API requests?
Key features:
- Prevent API abuse and DoS attacks
- Allow controlled access to APIs
- Implement rate limits per user, IP, or application
Design highlights:
- Use a Token Bucket Algorithm or Leaky Bucket Algorithm.
- Store rate limits in a distributed cache (Redis) for fast access.
- Implement exponential backoff for repeated violations.
Problem: How would you design a search system to handle billions of tweets and provide fast results?
Key features:
- Full-text search across tweets
- Real-time updates to indexed data
- Ranking tweets based on relevance
Design highlights:
- Use Elasticsearch for full-text search.
- Implement inverted indexes for efficient querying.
- Use sharding and partitioning to scale horizontally.
Problem: Design a system to efficiently distribute incoming network traffic across multiple servers.
Key features:
- Balance traffic based on requests per second (RPS)
- Perform regular health checks to identify and exclude failed servers
- Enable horizontal scaling to handle increased traffic
Design highlights:
- Utilize load balancing strategies like Round Robin or Least Connections.
- Deploy tools such as NGINX, HAProxy, or cloud-based load balancers.
- Apply consistent hashing to achieve more balanced and reliable traffic distribution.
- System design interviews at Twitter assess your ability to architect scalable, efficient, and reliable systems. These interviews focus on designing distributed systems, making trade-offs, and solving high-level technical challenges—key concepts covered in "Grokking the Modern System Design Interview."
Behavioral interview questions
Behavioral interviews at Twitter focus on problem-solving, collaboration, and leadership skills. Expect situational and experience-based questions. Below are examples of behavioral questions.
Q1. Tell me about a time you solved a complex problem.
Example answer: While working on a project, I noticed a high API latency affecting thousands of users. I analyzed the logs, found inefficient database queries, and optimized them using indexing and caching. This reduced response time by 60% and significantly improved user experience.
Q2. Describe a situation where you handled a conflict within a team.
Example answer: During a product discussion, a teammate and I disagreed on implementing a caching strategy. To resolve the conflict, we held a meeting, reviewed data from a small-scale test, and made an informed decision. This collaborative approach strengthened our team dynamics and improved system efficiency.
Q3. Tell me about a time when you led a project under tight deadlines.
Example answer: During a Twitter feature rollout, unexpected database issues arose. I quickly coordinated with engineers, implemented auto-scaling, and resolved the issue in 24 hours, ensuring a successful, on-time launch.
Q4. Give an example of when you had to convince others to adopt your idea.
Example answer: I proposed using GraphQL instead of REST APIs for an internal service, but my team was hesitant. I demonstrated the benefits through a small proof of concept, showing reduced API calls and improved efficiency. This convinced the team to adopt GraphQL.
Pro tip: Structure your responses using the STAR method (Situation, Task, Action, Result).
Q5. Describe how you handle feedback and criticism.
Example answer: During a code review at Twitter, a senior engineer pointed out that my feature implementation wasn’t scalable for future growth. Instead of taking it personally, I scheduled a quick follow-up to better understand the concerns. After the discussion, I refactored the code, applying a more modular design pattern. Not only did this make the feature scalable, but it also improved my coding practices. I appreciate constructive feedback because it helps me grow and deliver better solutions.
Q6. How do you ensure inclusivity and diversity in your work?
Example answer: In a previous project, I noticed that a few voices dominated team meetings while others, particularly remote teammates, rarely contributed. To address this, I suggested implementing structured agendas with dedicated time for everyone to share updates. I also encouraged asynchronous feedback through shared documents. This created a more inclusive environment where all perspectives were heard, leading to stronger solutions and higher team morale. I believe diverse input leads to better, more user-centered products.
- For tailored guidance on Twitter’s Leadership Principles and behavioral questions, explore Grokking the Behavioral Interview, which provides real-world examples to refine your responses effectively.
General tips to ace your Twitter interview
- Know Twitter’s products and features: Stay updated on Twitter Blue, Spaces, Communities, and X’s future vision.
- Understand Twitter’s business model: How does Twitter make money? What are its revenue streams?
- Practice behavioral questions: Use STAR format and demonstrate Twitter’s values.
- Prepare for technical rounds: For engineers, focus on data structures, algorithms, and system design.
- Follow Twitter trends: Be aware of recent news, platform updates, and controversies.
- Ask thoughtful questions: Show your enthusiasm by asking about team culture, challenges, and company goals.
Refine your skills with AI-powered mock interviews
Increase your chances of success with AI-driven mock interview platforms. These tools offer realistic coding, system design, and behavioral simulations, providing instant feedback to help you improve and refine your performance.
Recommended resources
- Grokking the Coding Interview Patterns: Learn 26 essential coding patterns to easily tackle thousands of LeetCode-style questions. Prepare efficiently for coding interviews with the ultimate course, designed by FAANG engineers.
- Grokking the Modern System Design Interview: The ultimate guide to System Design Interviews, crafted by FAANG engineers. Master distributed systems fundamentals and sharpen your skills with real-world interview questions and mock interviews.
- Grokking the Behavioral Interview: Whether you’re a software engineer, product manager, or engineering manager, this course equips you with the tools to confidently tackle behavioral and cultural questions. Beyond technical roles, it offers valuable insights for professionals in any field.
- Grokking the Low-Level Design Interview Using OOD Principles: A battle-tested guide to Object-Oriented Design (OOD) Interviews, crafted by FAANG engineers. Master OOD fundamentals and sharpen your skills with real-world interview questions.
- Grokking the Product Architecture Design Interview: The essential guide to API Design & Product Design Interviews – developed by FAANG engineers. Master product design fundamentals & get hands-on with real-world APIs.
Conclusion
Twitter interviews demand solid preparation in coding, system design, and behavioral alignment with the company’s fast-paced culture. Focus on data structures, algorithms, and real-world scalability challenges to excel in the technical rounds. System design expertise is crucial for mid-to-senior roles, so practice designing scalable architectures. Behavioral questions assess your collaboration, leadership, and adaptability, so prepare structured responses. Use LeetCode, Educative courses, and mock interviews to sharpen your problem-solving skills. With consistent practice and confidence, you’ll be well-prepared to land your dream job at Twitter!
Best of luck!
Frequently Asked Questions
What programming languages are preferred for Twitter interviews?
Twitter doesn’t mandate a specific language, but Python, Java, C++, and Scala are commonly used. Choose a language you’re most comfortable with, as clarity and efficiency matter more than the language itself.
Does Twitter ask system design questions for junior roles?
System design is more common for mid-level and senior roles, but juniors may get simplified questions. They might ask about designing a basic feature (e.g., a tweet storage system) rather than a full-scale distributed architecture.
How much does culture fit matter in Twitter interviews?
A lot! Twitter values inclusivity, innovation, and open communication. Expect behavioral questions that assess your alignment with their culture, such as how you collaborate, handle feedback, or contribute to a diverse team.
How long does the Twitter interview process take?
Depending on the role and team, it typically takes 3–6 weeks. The process includes an initial recruiter screen, technical rounds (coding + system design), and a final onsite with behavioral and leadership interviews.
How competitive is the Twitter interview process?
Twitter’s interviews are challenging but not impossible. They assess problem-solving skills, coding efficiency, system design expertise, and cultural fit. Strong preparation in algorithms, real-world architecture, and communication will give you an edge.
Company Interview Questions